5 Must GitHub Checks for a Python Repo
Solutions to ensure your code always measures up to the programming standards
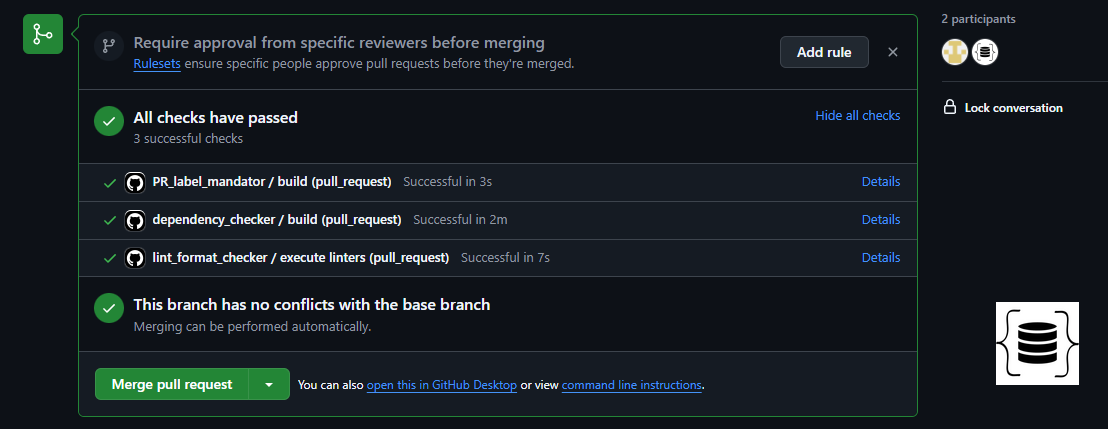
Version control systems (VCS) are the source of truth for the code.
Change or commit history and code lineage features of VSC make it stand out in terms of its uses.
As part of the software development life cycle, every new feature, integration, bug fix, and improvement has to be merged into the code repo.
These code repos act as bridges between tools and services, enabling the desired functionality.
For example, CI/CD pipelines or automated scripts will clone the approved repos versions onto clusters for execution.
It is increasingly important for the developer or maintainer to ensure the code complies with certain checks before it is merged into the main/master branch.
Your level as a developer/programmer doesn't matter when it comes to GitHub checks.
A miss is a miss; there is no way around it unless you fix it and recommit the highlighted issue.
I am assuming you know how to create GitHub actions. We will set up 5 necessary and effective checks on our repo. If not, please refer to the video below.
These checks must be successfully passed before merging the code from the feature to the main or master branch.
Check 1: Are Library Dependencies Safe to Use?
Software is made possible because of the diverse ecosystem and contributors offering a variety of language-specific libraries that solve or address a specific problem.
Library dependencies can easily influence the software program’s functionality.
Python for example has a vibrant ecosystem with thousands of open-source libraries.
Every library evolves, adding new features and fixing issues reported by users as bugs. These can be breaking changes.
Breaking changes can impact the performance, jeopardize security, introduce vulnerabilities, mess up the functionality, and much more.
From a security standpoint, checking if any library or dependency imposes a security vulnerability is crucial.
We will set up our first check to scan and audit all the dependent libraries used in the current build.
Since our focus is on Python, pip-audit
is a sound choice to scan for vulnerabilities.
When a developer pushes code to the feature branch and creates a pull request for code merge.
The dependency check will install all the required libraries from the requirements.txt file and run pip-audit
to scan for vulnerabilities.
#@toolexi / @Databracket
name: dependency_checker
on:
pull_request:
branches: [ "main" ]
workflow_dispatch:
jobs:
build:
runs-on: ubuntu-latest
steps:
- name: repository checkout
uses: actions/checkout@v4
- name: python setup
uses: actions/setup-python@v4
with:
python-version: '3.9'
- name: Dependency install
run: |
python -m venv venv_toolexi
source venv_toolexi/bin/activate
pip install -r requirements.txt
- name: Install pip-audit
run: pip install pip-audit
- name: Run pip-audit
run: pip-audit
Only successful checks allow the PR to merge.
If a failure occurs, flagging vulnerabilities in any of the libraries, the developer must fix the issue before merging the code.
Additionally, we can enable the inbuilt Github settings to ensure the dependencies are secure and updated.
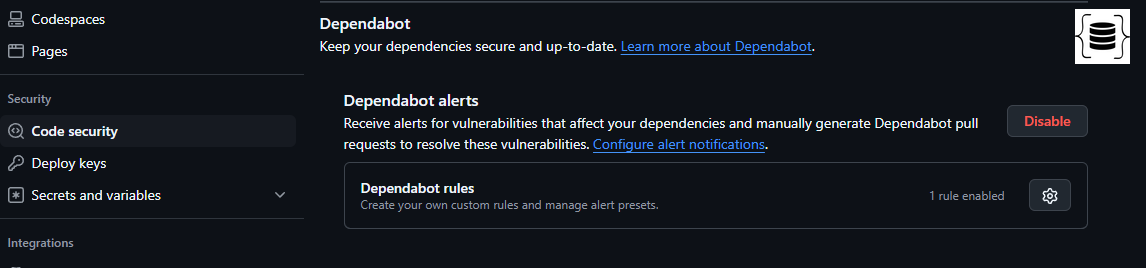
This places the business or organization in a secure spot from leaks and security threats.
Check 2: Secrets and Configs Exposure
Logical coordination between various software services and applications is required to deliver desired functionalities.
These logical components bi-directionally share data and functionality, ensuring the flow remains stagnant.
These connectivities require secret tokens, configurations, and passwords for authentication and authorization.
Vaults are the go-to option for organizations to store and retrieve secrets.
Sometimes, developers end up passing secrets inline or from config files, and unknowingly they will push the code to Github.
These secrets get exposed, and everyone with reader access to the repository can view the secrets.
Internal teams tend to ignore these secrets, but having secrets in a central code repository is not a good practice.
Once committed, we can revert the changes from the main or master branch, but the secrets will still be present in the version history.
#@toolexi / @Databracket
name: secrets_scanner
on:
push
pull_request:
branches: [ "main" ]
jobs:
scan-secrets:
name: scanning for secrets
runs-on: ubuntu-latest
steps:
- name: repository checkout
uses: actions/checkout@v3
- name: Secret scanning action
uses: secret-scanner/action@0.0.2
This can be avoided by enabling a simple Github setting that automatically scans for secrets in the entire codebase and reports if found.
Additionally, we can enable a GitHub action that blocks the PR merge if any secrets are found in the code.
Check 3 and 4: Lint and Code formatting.
Code readability and formatting are important when dealing with a large code base.
Unstructured and undocumented code makes it increasingly difficult to understand and backtrace the functionality for a new set of eyes.
We can apply lint and code formatting tools to ensure the committed code is syntactically and structurally correct.
We can use flake8 black
libraries to enable lint and format checks in GitHub.
name: lint_format_checker
on:
push:
branches: [ "main" ]
pull_request:
branches: [ "main" ]
workflow_dispatch:
jobs:
lint-checker:
name: execute linters
runs-on: ubuntu-latest
steps:
- name: Check out Git repository
uses: actions/checkout@v4
- name: Set up Python
uses: actions/setup-python@v4
with:
python-version: 3.9
- name: Install flake8 and black
run: pip install flake8 black
- name: Run linters
run: flake8 .
- name: Run formatter
run: black --check .
The yaml config will ensure the libraries are installed and executed during the PR merge.
When the checks pass, the PR merge will be enabled.
Check 5: Enable Tagging or Labels
In the end, it boils down to stats and metrics.
How much did an individual developer and a team contribute to the development process?
We can mandate labels for PRs so that changes can be programmatically tracked and measured.
name: PR_label_mandator
on:
pull_request:
branches: [ "main" ]
workflow_dispatch:
jobs:
build:
runs-on: ubuntu-latest
steps:
- name: Check for the label
uses: actions/github-script@v5
with:
script: |
const labels = context.payload.pull_request.labels;
if (labels.length === 0) {
core.setFailed("Label is required to merge this pull request")
} else {
labels.forEach(label => {
console.log(`Label "${label.name}" is added to the PR.`);
});
}
This action is unique from other actions we saw already.
Here we can apply custom logic to enhance the overall behavior and check.
Final Thoughts
Software is emerging rapidly and taking over the world. Software has the potential to tap real-world problems and offer solutions at scale.
When building consumer-facing or enterprise applications, code quality and security are important.
Checking every part of the software can be a time-consuming endeavor. The version control system can bridge these manual checks by allowing us to enable automated code or process checks.
These checks ensure the code complies with all the mandatory requirements and security standards.
Connect with Me
📰 Linkedin | 🐦Twitter | 📽️Youtube | 📖 Medium | 🪝 Gumroad | 🧑💻Github | 📷Instagram